Landing a job in the automotive tech industry, especially in roles involving software and data, often hinges on how well you perform in coding interviews. Companies in the car industry, from established manufacturers to innovative tech startups focused on electric vehicles and autonomous driving, are increasingly relying on data-driven decisions. This means that demonstrating your coding skills is crucial.
To help you navigate this crucial step, we’ve compiled a set of coding interview questions relevant to the car industry. These questions, inspired by real-world scenarios in companies like Tesla and others in the automotive sector, will prepare you to impress your interviewers.
11 Car Coding Interview Questions to Practice
While SQL is prominently featured in the original article, remember that car coding interviews can span various programming languages and problem types. However, given the prevalence of data analysis in the automotive industry, SQL remains a highly relevant skill.
Coding Question 1: Analyzing Electric Vehicle Charging Station Usage with SQL
Imagine you’re working with data from a network of electric vehicle charging stations. To understand usage patterns, you need to analyze charging session data. The dataset includes timestamps for when a car starts and finishes charging, along with the station ID. Your task is to calculate the total charging time for each station and compare it to the previous day’s usage.
Example Input:
charge_id | start_time | end_time | station_id | car_id |
---|---|---|---|---|
1001 | 07/01/2022 08:00:00 | 07/01/2022 09:00:00 | 2001 | 3001 |
1002 | 07/01/2022 12:00:00 | 07/01/2022 13:00:00 | 2001 | 3002 |
1003 | 07/02/2022 10:00:00 | 07/02/2022 11:00:00 | 2002 | 3003 |
1004 | 07/02/2022 11:30:00 | 07/02/2022 12:30:00 | 2001 | 3001 |
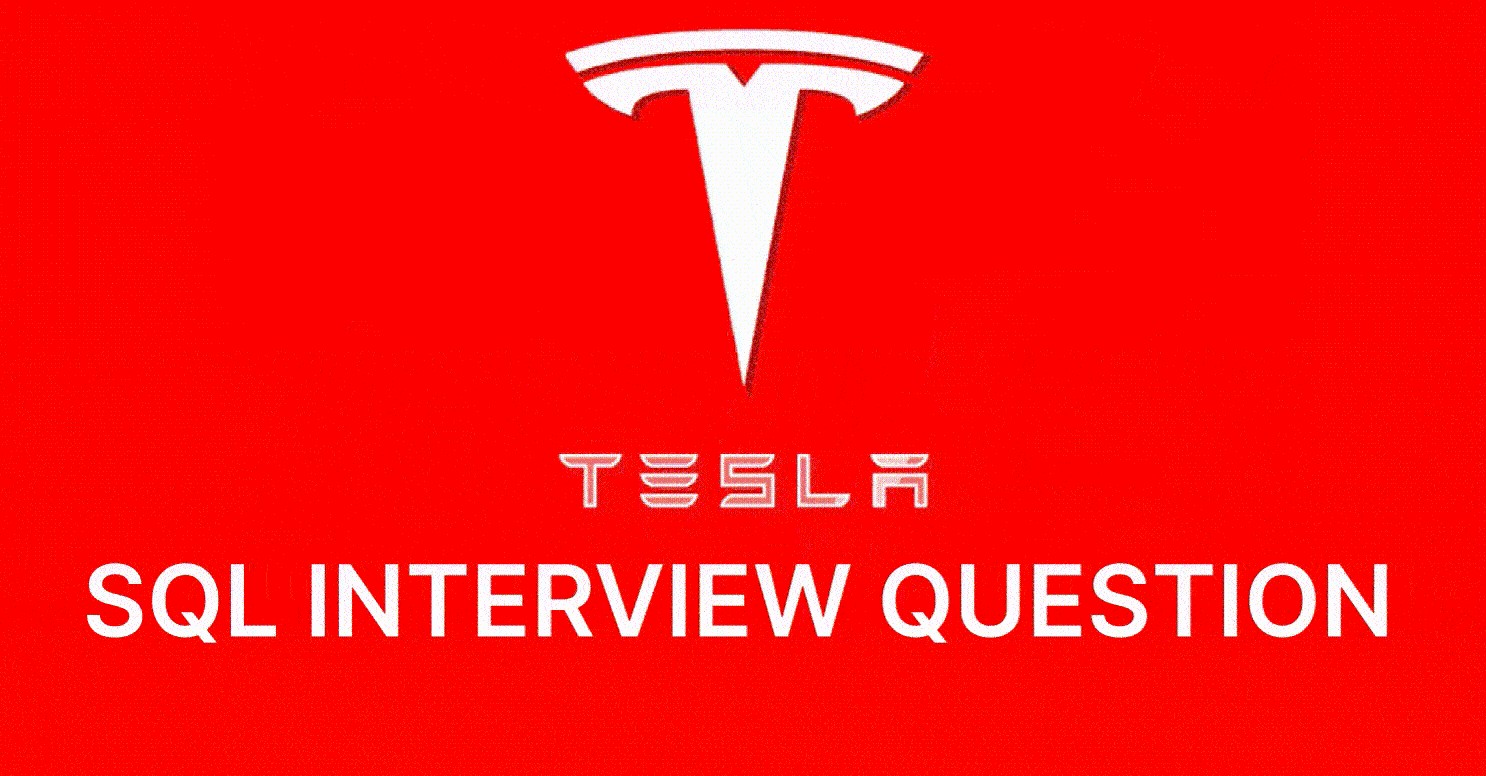
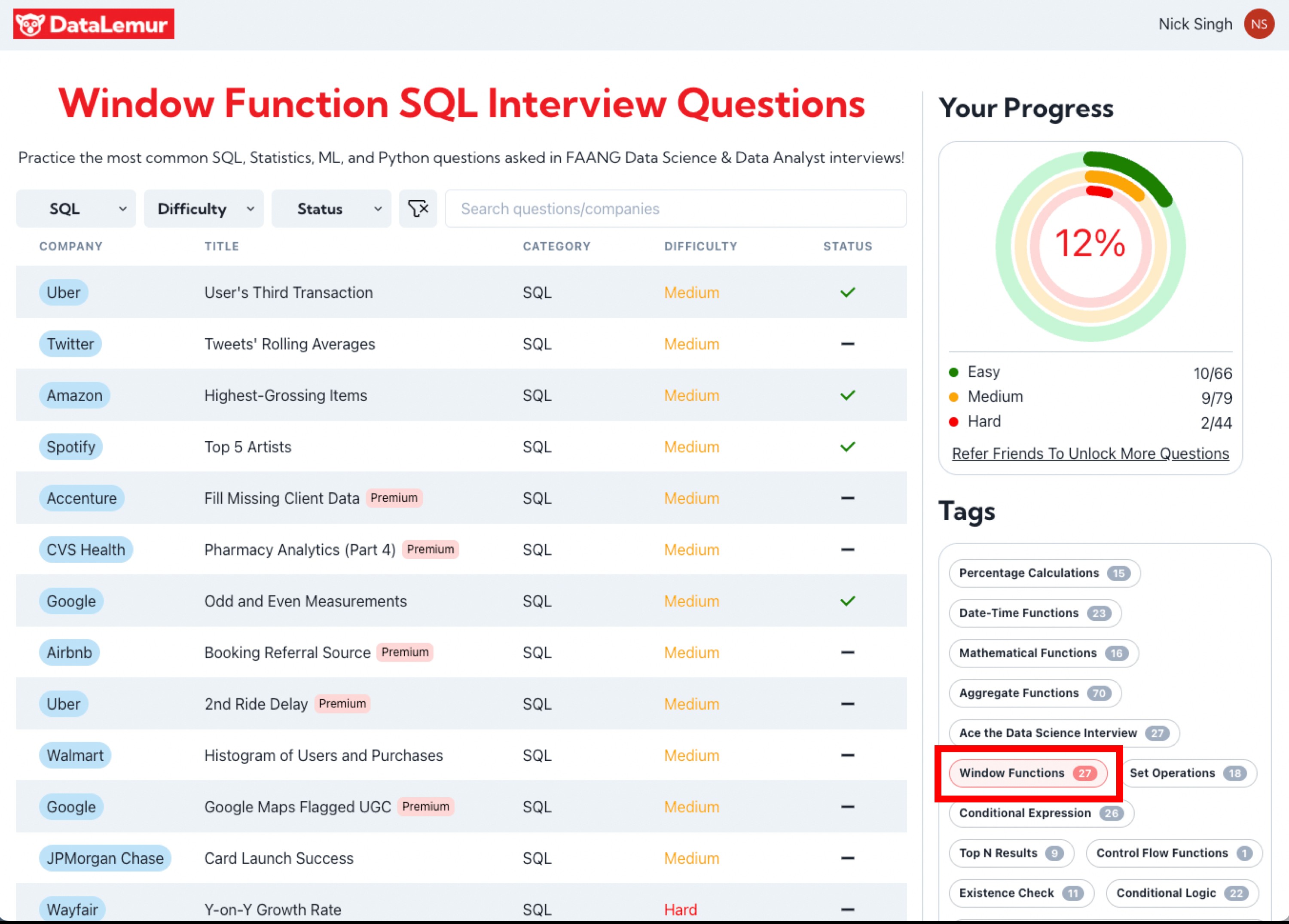
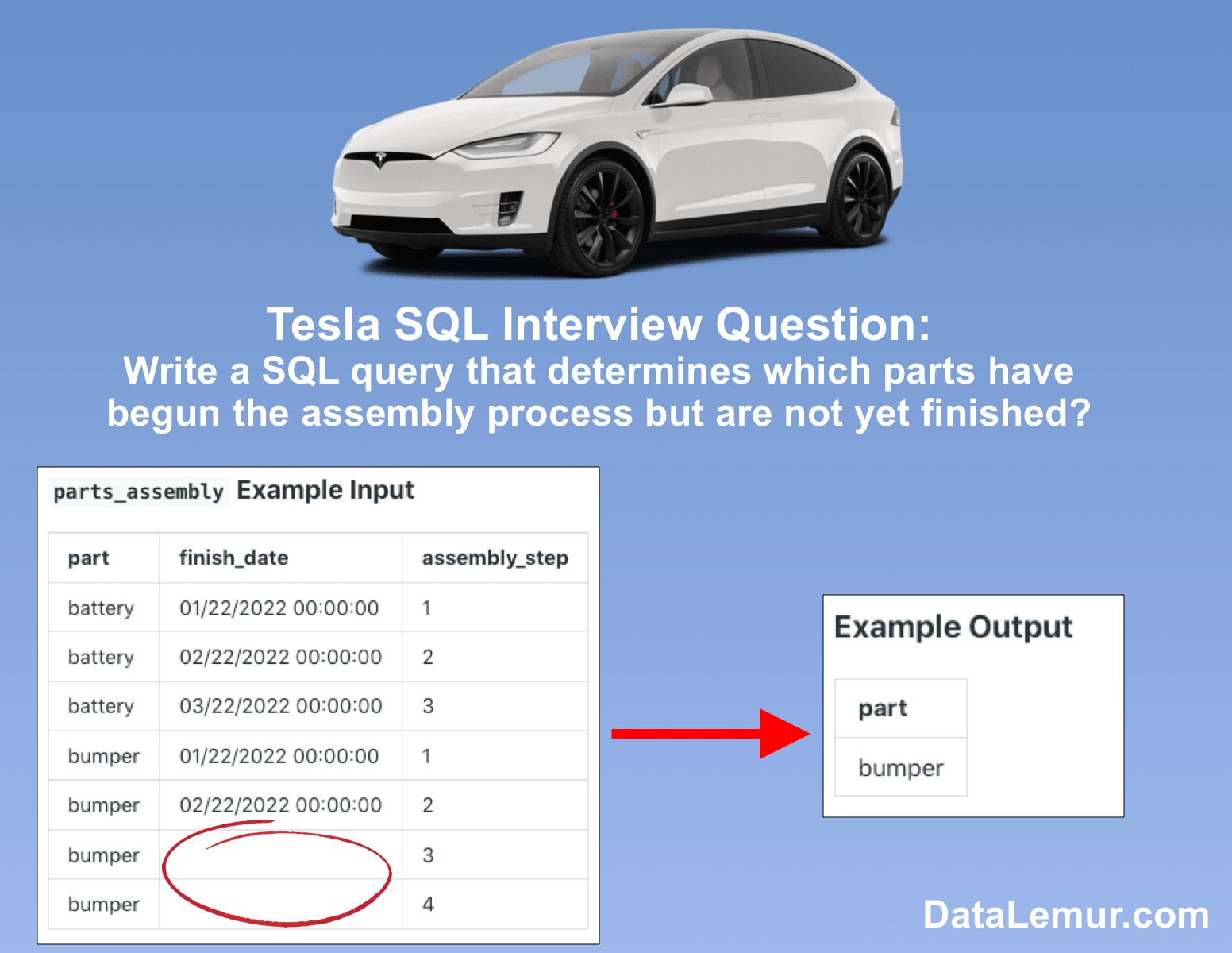
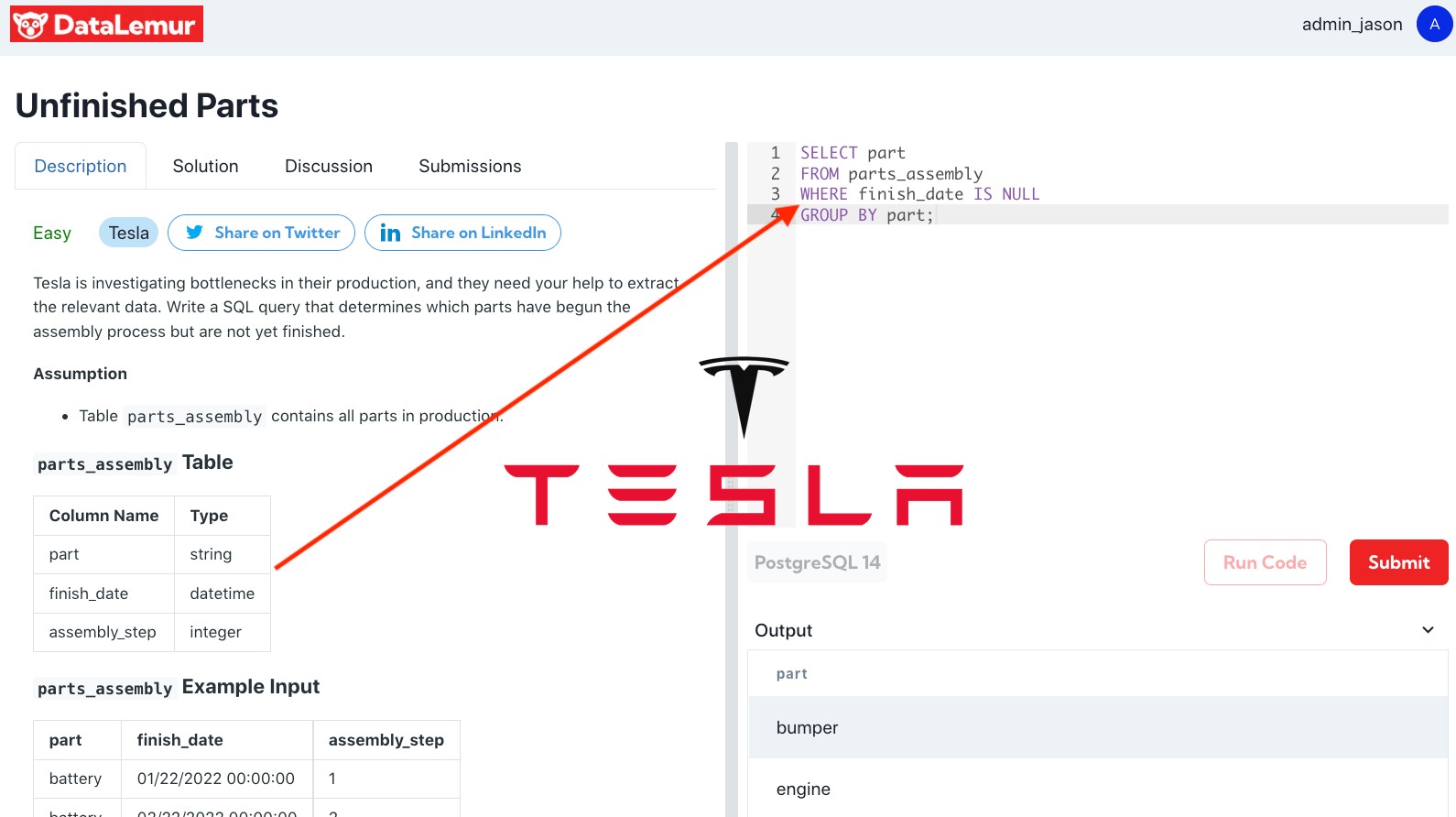
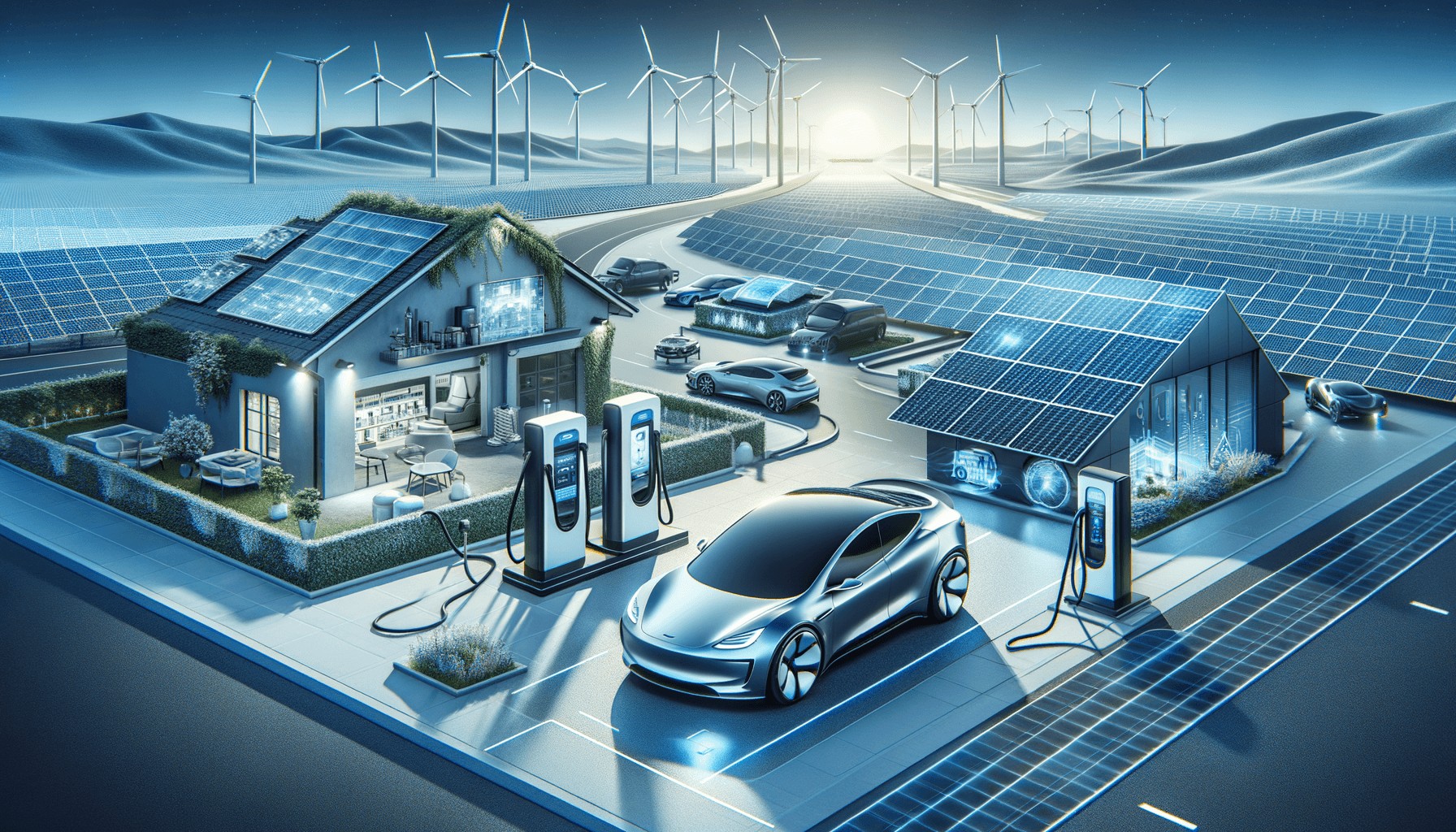
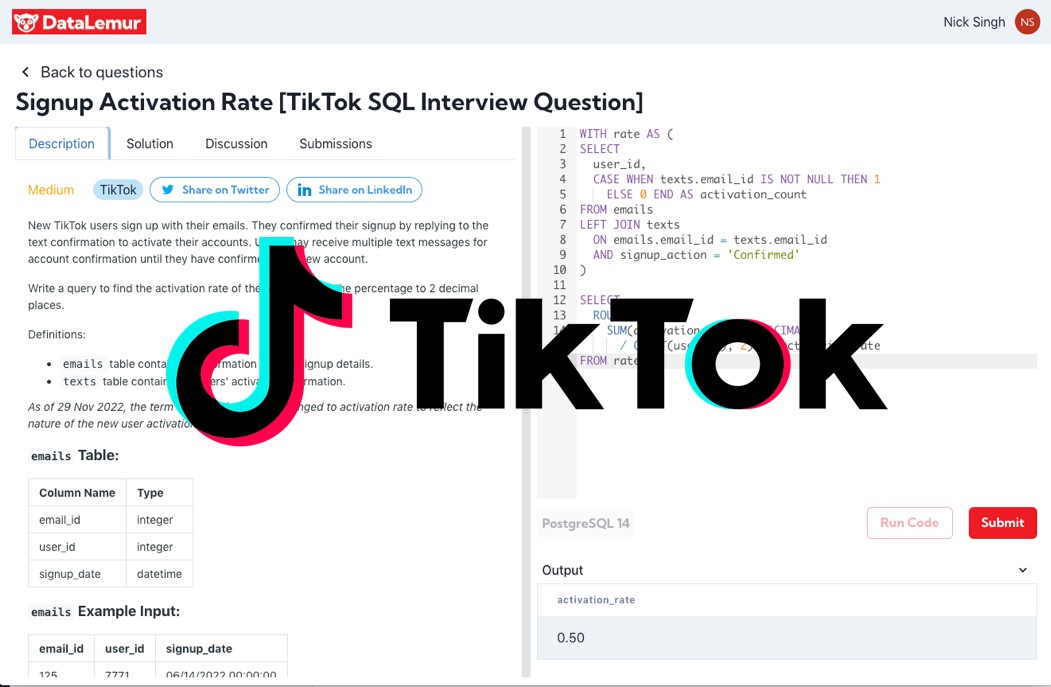
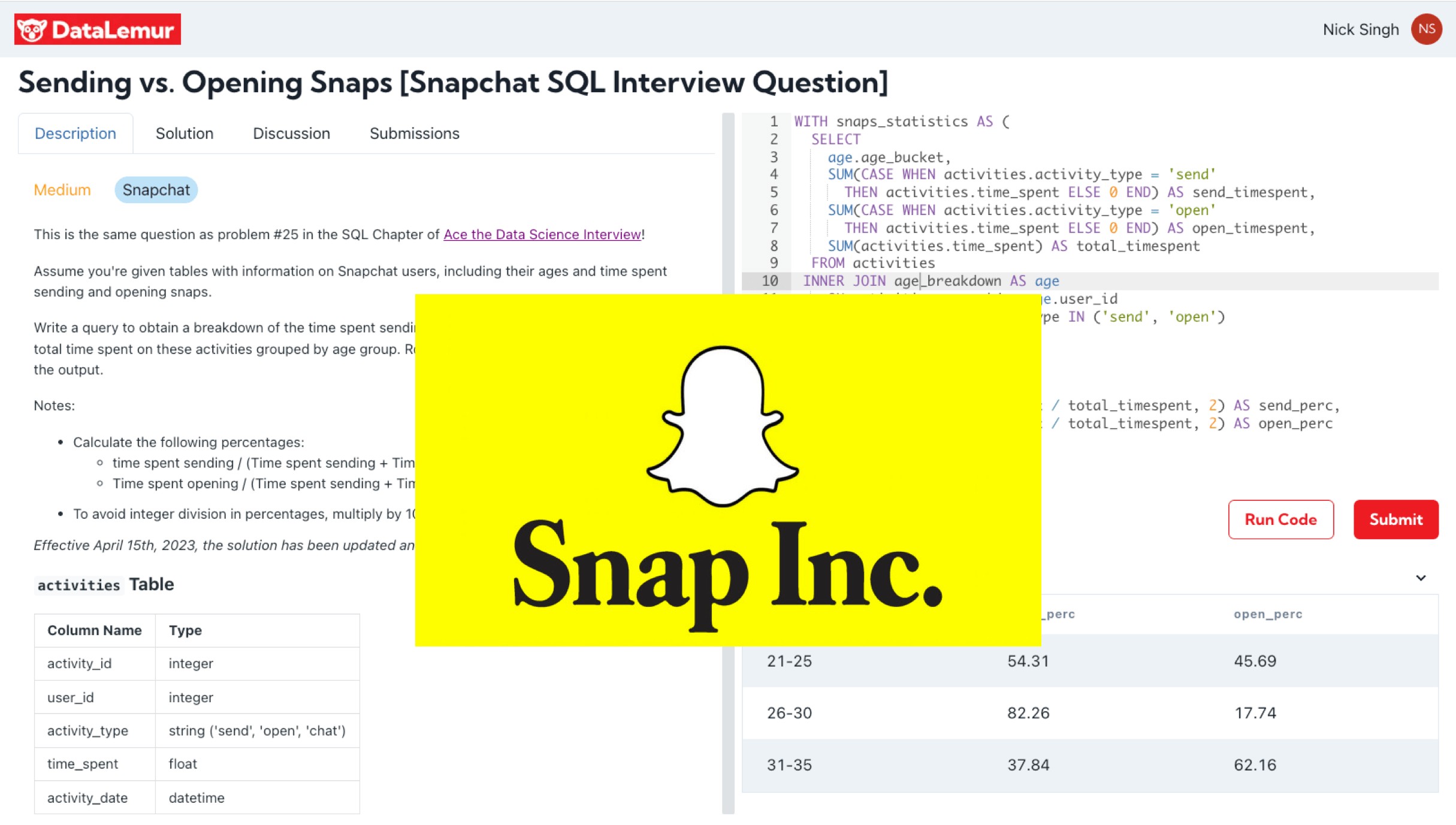
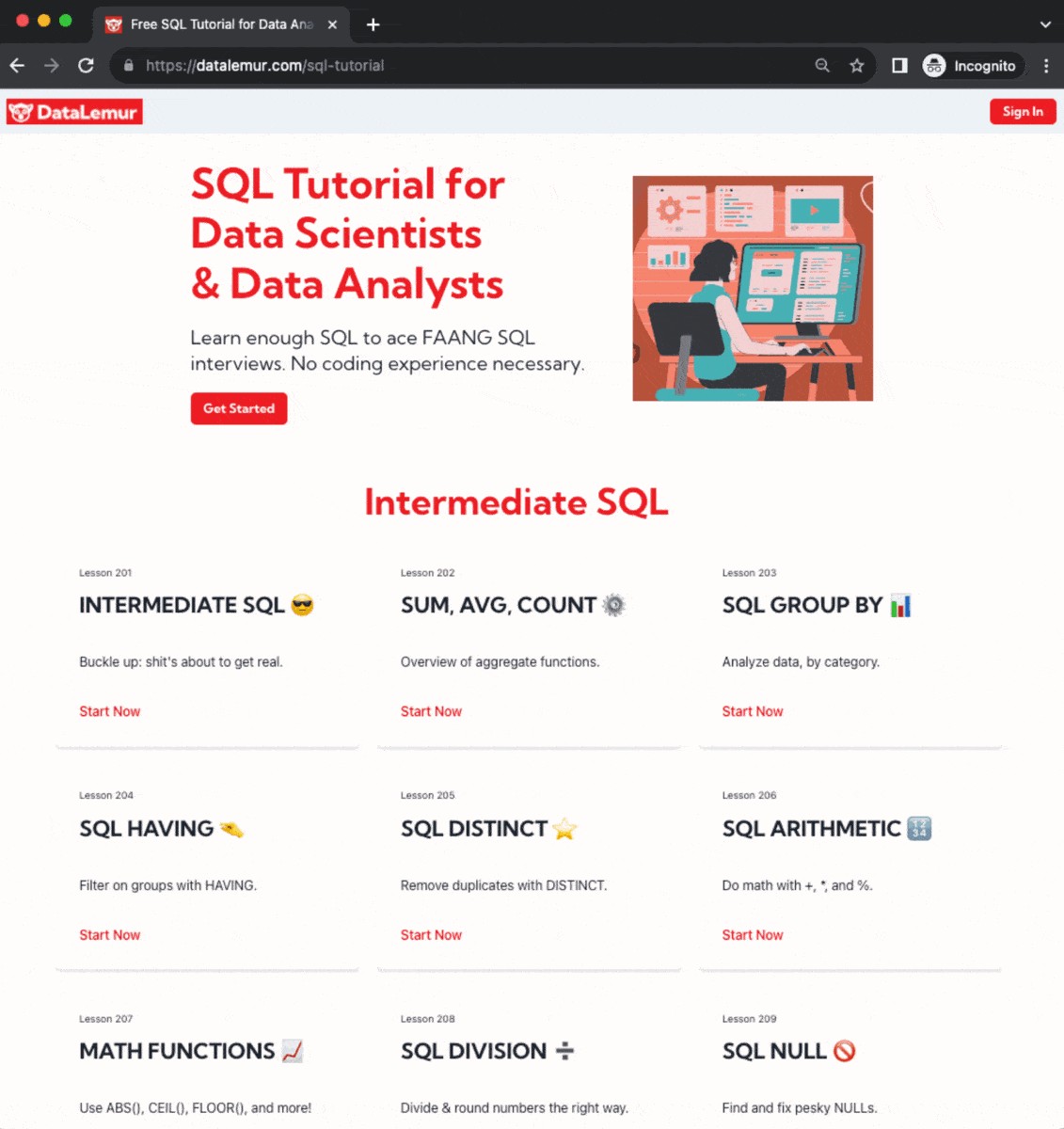
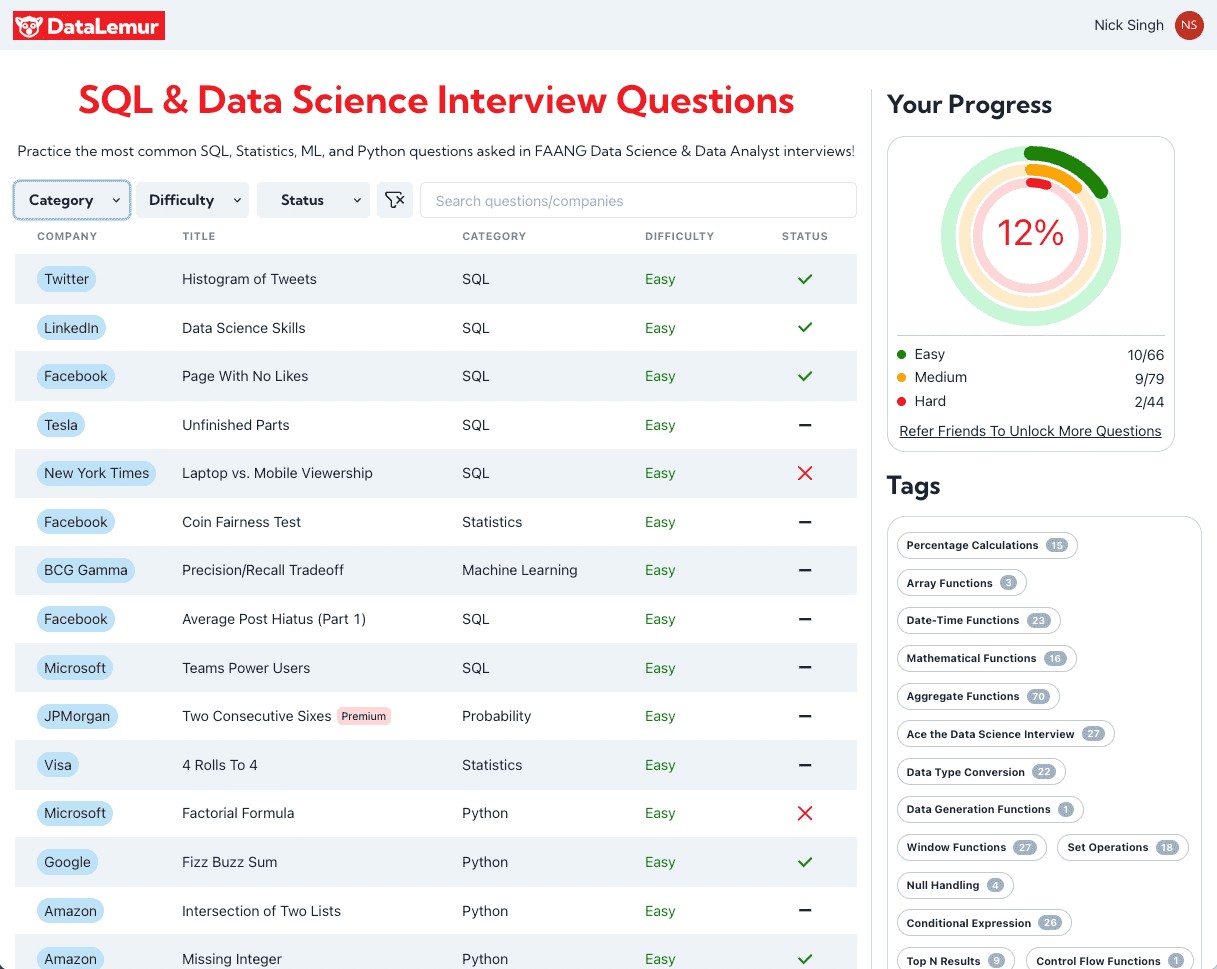
This table shows charging session details, including start and end times, station IDs, and car IDs.
SQL Answer:
WITH DailyStationUsage AS (
SELECT
station_id,
DATE(start_time) AS charging_date,
SUM(EXTRACT(EPOCH FROM (end_time - start_time))) / 3600 AS total_charging_hours
FROM
charging_sessions
GROUP BY
station_id, DATE(start_time)
)
SELECT
station_id,
charging_date,
total_charging_hours,
LAG(total_charging_hours, 1, 0) OVER (PARTITION BY station_id ORDER BY charging_date) AS previous_day_charging_hours,
total_charging_hours - LAG(total_charging_hours, 1, 0) OVER (PARTITION BY station_id ORDER BY charging_date) AS charging_hour_difference
FROM
DailyStationUsage
ORDER BY
station_id, charging_date;
This SQL query uses window functions to compare daily charging times. It calculates the total charging hours for each station per day and then uses the LAG()
function to access the previous day’s total, allowing for easy comparison. Understanding window functions is key for efficient data analysis in SQL, a skill highly valued in car tech companies dealing with large datasets.
Coding Question 2: Identifying Unfinished Car Parts in Production (SQL)
Car manufacturing involves complex assembly processes. Imagine you have a database table tracking car parts in production. A real-world coding interview question might ask you to identify parts that have started assembly but are not yet marked as finished.
Given a table named parts_production
with columns like part_id
and finish_date
, write a SQL query to find parts that have begun production (implying they exist in the table) but are missing a finish_date
.
SQL Answer:
SELECT
part_id
FROM
parts_production
WHERE
finish_date IS NULL;
This simple SQL query effectively identifies unfinished parts by selecting part_id
where the finish_date
is null, indicating the assembly process is ongoing. This type of question tests your ability to understand data representation and use SQL for practical manufacturing process analysis.
Coding Question 3: Understanding SQL Constraints – The CHECK Constraint
Beyond writing queries, coding interviews may also assess your understanding of database concepts. A question might ask: “How does the CHECK
constraint in SQL function, and in what scenarios is it useful in the context of car data?”
The CHECK
constraint enforces data integrity by ensuring that values in a column meet specific conditions.
Answer:
The CHECK
constraint is crucial for maintaining data quality in car industry databases. For example:
-
Vehicle Speed: Ensuring a
speed
column in a vehicle telematics table only accepts positive values:ALTER TABLE vehicle_telemetry ADD CONSTRAINT ck_speed CHECK (speed >= 0);
-
Battery Voltage: Validating that
battery_voltage
is within an acceptable range:ALTER TABLE ev_battery_data ADD CONSTRAINT ck_battery_voltage CHECK (battery_voltage BETWEEN 200 AND 800);
Using CHECK
constraints helps prevent invalid data entry, ensuring the reliability of data used for analysis and decision-making in car development and manufacturing.
Coding Question 4: Calculating Conversion Rates for Car Model Digital Ads (SQL)
Marketing and sales are vital in the car industry. Suppose you need to analyze the effectiveness of digital ad campaigns for different car models. You have data on ad clicks and website cart additions. The task is to calculate the click-through conversion rate for each ad campaign and car model.
Example Input: ad_clicks
table
click_id | user_id | click_date | ad_campaign | product_model |
---|---|---|---|---|
1256 | 867 | 06/08/2022 00:00:00 | Campaign1 | Model S |
2453 | 345 | 06/08/2022 00:00:00 | Campaign2 | Model X |
4869 | 543 | 06/10/2022 00:00:00 | Campaign1 | Model 3 |
7853 | 543 | 06/18/2022 00:00:00 | Campaign3 | Model Y |
3248 | 865 | 07/26/2022 00:00:00 | Campaign2 | Model S |
Example Input: add_to_carts
table
cart_id | user_id | add_date | product_model |
---|---|---|---|
1234 | 867 | 06/08/2022 00:00:00 | Model S |
7324 | 345 | 06/10/2022 00:00:00 | Model X |
6271 | 543 | 06/11/2022 00:00:00 | Model 3 |
SQL Answer:
WITH AdClicks AS (
SELECT
ad_campaign,
product_model,
COUNT(DISTINCT user_id) AS click_count
FROM
ad_clicks
GROUP BY
ad_campaign, product_model
),
AddToCart AS (
SELECT
product_model,
COUNT(DISTINCT user_id) AS cart_add_count
FROM
add_to_carts
GROUP BY
product_model
)
SELECT
ac.ad_campaign,
ac.product_model,
(CASE WHEN ac.click_count = 0 THEN 0 ELSE (CAST(COALESCE(atc.cart_add_count, 0) AS FLOAT) / ac.click_count) * 100 END) AS conversion_rate
FROM
AdClicks ac
LEFT JOIN
AddToCart atc ON ac.product_model = atc.product_model
ORDER BY
ac.ad_campaign, ac.product_model;
This SQL query calculates conversion rates by joining two CTEs (Common Table Expressions). AdClicks
counts clicks per campaign and model, and AddToCart
counts cart additions per model. The final SELECT statement joins these and calculates the conversion rate as a percentage of clicks that resulted in cart additions. Analyzing marketing data is crucial for optimizing car sales strategies.
Coding Question 5: Self-Joins for Website Navigation Analysis (SQL)
Understanding user behavior on car manufacturer websites is key to improving user experience. A coding interview question might involve analyzing website navigation paths. “What is a self-join in SQL, and how could you use it to analyze website page referrals on a car website?”
A self-join involves joining a table to itself, useful for comparing rows within the same table.
Answer:
Consider website visitor data with page URLs and referrer URLs. To find pairs of pages where one page refers to another on a car website, you can use a self-join:
SELECT
p1.url AS current_page_url,
p2.url AS referrer_page_url
FROM
website_pages AS p1
JOIN
website_pages AS p2 ON p1.referrer_id = p2.page_id
WHERE p1.page_id != p2.page_id; -- Exclude self-referrals
This query joins the website_pages
table to itself, aliased as p1
and p2
. It links rows where p1.referrer_id
matches p2.page_id
, effectively showing page referrals. Self-joins are powerful for analyzing relationships within a single dataset, like website navigation or social networks.
Coding Question 6: Average Car Selling Price Trend Analysis Over Years (SQL)
Sales data analysis is fundamental for car companies. Imagine you need to track the average selling price of each car model over different years to identify market trends. Write a SQL query to calculate the average selling price per car model for each year, given a sales data table.
Example Input:
sale_id | model_id | sale_date | price |
---|---|---|---|
1 | ModelS | 2018-06-08 | 80000 |
2 | ModelS | 2018-10-12 | 79000 |
3 | ModelX | 2019-09-18 | 100000 |
4 | Model3 | 2020-07-26 | 38000 |
5 | Model3 | 2020-12-05 | 40000 |
6 | ModelY | 2021-06-08 | 50000 |
7 | ModelY | 2021-10-10 | 52000 |
SQL Answer:
SELECT
EXTRACT(YEAR FROM sale_date) AS sale_year,
model_id,
AVG(price) AS average_price
FROM
sales_data
GROUP BY
sale_year, model_id
ORDER BY
sale_year, model_id;
This SQL query extracts the year from the sale_date
, then groups the data by year and model_id
to calculate the average price for each car model in each year. Analyzing sales trends over time is critical for strategic decision-making in the automotive industry.
Coding Question 7: Clustered vs. Non-Clustered Indexes in Car Databases
Database performance is crucial, especially with the massive data volumes in modern cars. You might be asked to explain the differences between clustered and non-clustered indexes in SQL and their implications for car data management.
Answer:
-
Clustered Index: Physically orders the data rows in the table based on the index key. A table can have only one clustered index. Think of it like a phone book where entries are physically ordered by last name. This is beneficial for range queries and ordered data retrieval, useful for time-series car data like sensor readings.
-
Non-Clustered Index: Creates a separate index structure that points to the actual data rows. A table can have multiple non-clustered indexes. Like a book index, it allows for faster lookups without reordering the physical data. Useful for frequently queried columns that are not used for physical ordering, such as vehicle VIN or owner ID.
Choosing the right type of index impacts query performance significantly, especially when dealing with large automotive datasets.
Coding Question 8: Joining Customer and Car Information (SQL)
Connecting customer data with vehicle information is essential for CRM and personalized services in the car industry. Given two tables, customers
and cars
, design a SQL query to retrieve customer details along with the car they purchased.
Example Input: customers
table
customerID | name | purchase_date | |
---|---|---|---|
1 | John Doe | [email protected] | 2022-01-01 |
2 | Jane Smith | [email protected] | 2022-04-12 |
3 | Mike Thomas | [email protected] | 2022-06-20 |
Example Input: cars
table
carID | model | color | customerID |
---|---|---|---|
1 | Model S | Blue | 1 |
2 | Model X | White | 2 |
3 | Model 3 | Black | 3 |
SQL Answer:
SELECT
c.name,
c.email,
car.model,
car.color
FROM
customers c
JOIN
cars car ON c.customerID = car.customerID;
This SQL query uses a JOIN
clause to combine the customers
and cars
tables based on the common customerID
column. It retrieves customer names, emails, and the model and color of the car they purchased. Joins are fundamental for combining related data across tables in relational databases, a common task in car industry data management.
Coding Question 9: Calculating Battery Performance Index (SQL)
Battery efficiency is a critical metric for electric vehicle performance. Suppose you have test data for EV batteries, including charge and discharge energy and test duration. Calculate a battery performance index using the formula: Performance Index = ABS(CHARGE - DISCHARGE) / SQRT(DAYS)
. Round the result to two decimal places.
Sample Input:
run_id | battery_model | start_date | end_date | charge_energy | discharge_energy |
---|---|---|---|---|---|
1 | Model S | 2021-07-31 | 2021-08-05 | 100 | 98 |
2 | Model S | 2021-08-10 | 2021-08-12 | 102 | 99 |
3 | Model 3 | 2021-09-01 | 2021-09-04 | 105 | 103 |
4 | Model X | 2021-10-01 | 2021-10-10 | 110 | 107 |
5 | Model 3 | 2021-11-01 | 2021-11-03 | 100 | 95 |
SQL Answer:
SELECT
run_id,
battery_model,
ROUND(ABS(charge_energy - discharge_energy) / SQRT(DATE(end_date) - DATE(start_date) + 1), 2) AS performance_index
FROM
battery_tests;
This SQL query calculates the battery performance index according to the given formula. It uses ABS()
for the absolute difference, SQRT()
for the square root of test duration in days, and ROUND()
to format the result to two decimal places. Performance metrics are vital for evaluating and improving car components like batteries.
Coding Question 10: SQL Constraints – Explanation and Use Cases in Car Data
Expect conceptual questions beyond just writing queries. “Can you explain SQL constraints and why they are useful, especially when managing car-related data?”
Answer:
SQL constraints are rules enforced on data within a database. They ensure data integrity and consistency. In the context of car data, examples include:
NOT NULL
: Ensuring critical fields likeVIN
(Vehicle Identification Number) orengine_serial_number
are always populated.UNIQUE
: Guaranteeing thatVIN
s andcustomer_ids
are unique within their respective tables.PRIMARY KEY
: Identifying unique records, like usingVIN
as the primary key for avehicles
table.FOREIGN KEY
: Establishing relationships between tables, e.g., linkingcustomer_id
in avehicles
table tocustomer_id
in acustomers
table to track vehicle ownership.CHECK
: Validating data values, like ensuringvehicle_speed
is always positive, as discussed in Question 3.DEFAULT
: Setting default values, such as settingregistration_date
to the current date when a new vehicle record is created.
Constraints are essential for building robust and reliable car data management systems.
Coding Question 11: Analyzing Vehicle Fleet Efficiency (SQL)
For companies managing car fleets, efficiency analysis is crucial. Suppose you have two tables: vehicles
(vehicle information) and service_records
(service data including distance driven and power consumed). Write a SQL query to report the average distance driven and average power consumed for each car model manufactured in each year.
Example Input: vehicles
table
vehicle_id | model_name | manufacture_year | owner_id |
---|---|---|---|
001 | Model S | 2018 | 1001 |
002 | Model 3 | 2019 | 1002 |
003 | Model X | 2020 | 1003 |
004 | Model S | 2019 | 1004 |
005 | Model 3 | 2018 | 1005 |
Example Input: service_records
table
record_id | vehicle_id | distance_driven | power_consumed |
---|---|---|---|
a001 | 001 | 1200 | 400 |
a002 | 002 | 1000 | 250 |
a003 | 003 | 1500 | 500 |
a004 | 001 | 1300 | 450 |
a005 | 004 | 1100 | 420 |
SQL Answer:
SELECT
v.model_name,
v.manufacture_year,
AVG(sr.distance_driven) AS avg_distance_driven,
AVG(sr.power_consumed) AS avg_power_consumed
FROM
vehicles v
JOIN
service_records sr ON v.vehicle_id = sr.vehicle_id
GROUP BY
v.model_name, v.manufacture_year
ORDER BY
v.model_name, v.manufacture_year;
This SQL query joins vehicles
and service_records
tables on vehicle_id
. It groups the results by model_name
and manufacture_year
to calculate the average distance driven and power consumed for each model and year. Fleet efficiency analysis helps optimize operations and reduce costs for car fleet operators.
Preparing for Your Car Coding Interview
Practice is paramount for success in coding interviews. Beyond these car-focused questions, expand your practice to cover a wide range of coding problems, data structures, and algorithms.
To further enhance your preparation:
- Practice SQL extensively: Focus on window functions, joins, aggregations, and constraints. Platforms like DataLemur offer hundreds of SQL practice questions from real companies.
- Master Data Structures and Algorithms: Be comfortable with arrays, linked lists, trees, graphs, sorting, searching, and dynamic programming.
- Review Object-Oriented Programming (OOP) concepts: Especially if applying for software engineering roles.
- Understand Car Industry Fundamentals: Familiarize yourself with basic car systems, electric vehicles, autonomous driving concepts, and industry trends. This context will help you understand the relevance of coding questions.
- Practice with Different Programming Languages: While SQL is vital for data roles, be proficient in languages like Python, Java, or C++, depending on the job requirements.
By combining focused practice with an understanding of the car industry, you’ll be well-equipped to ace your car coding interviews and drive your career forward in this exciting field.